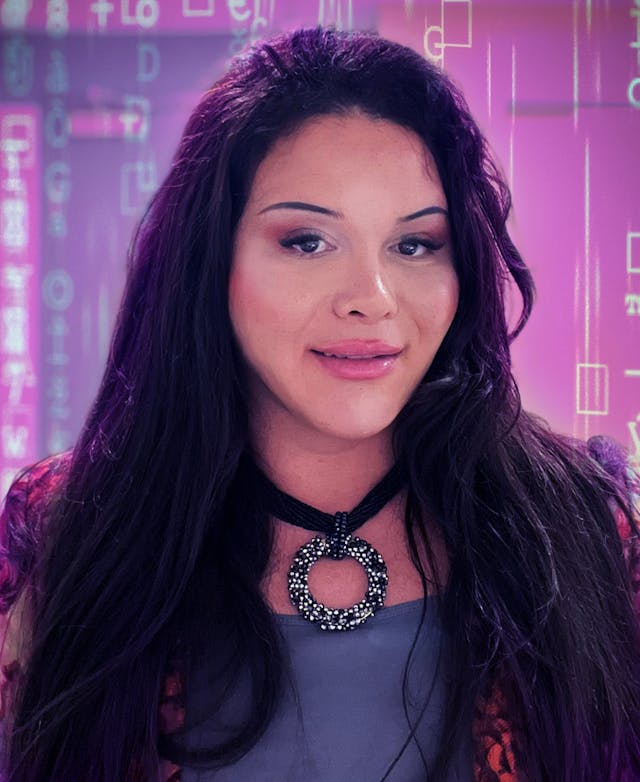
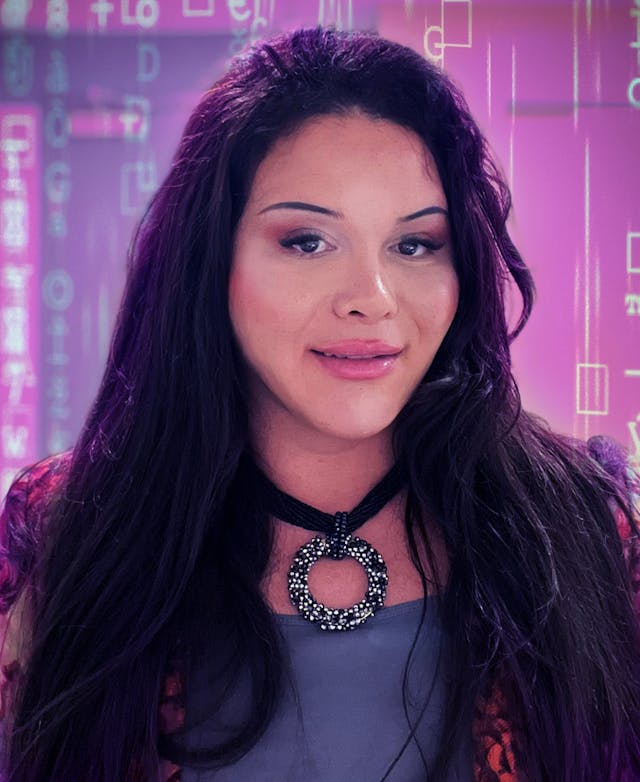
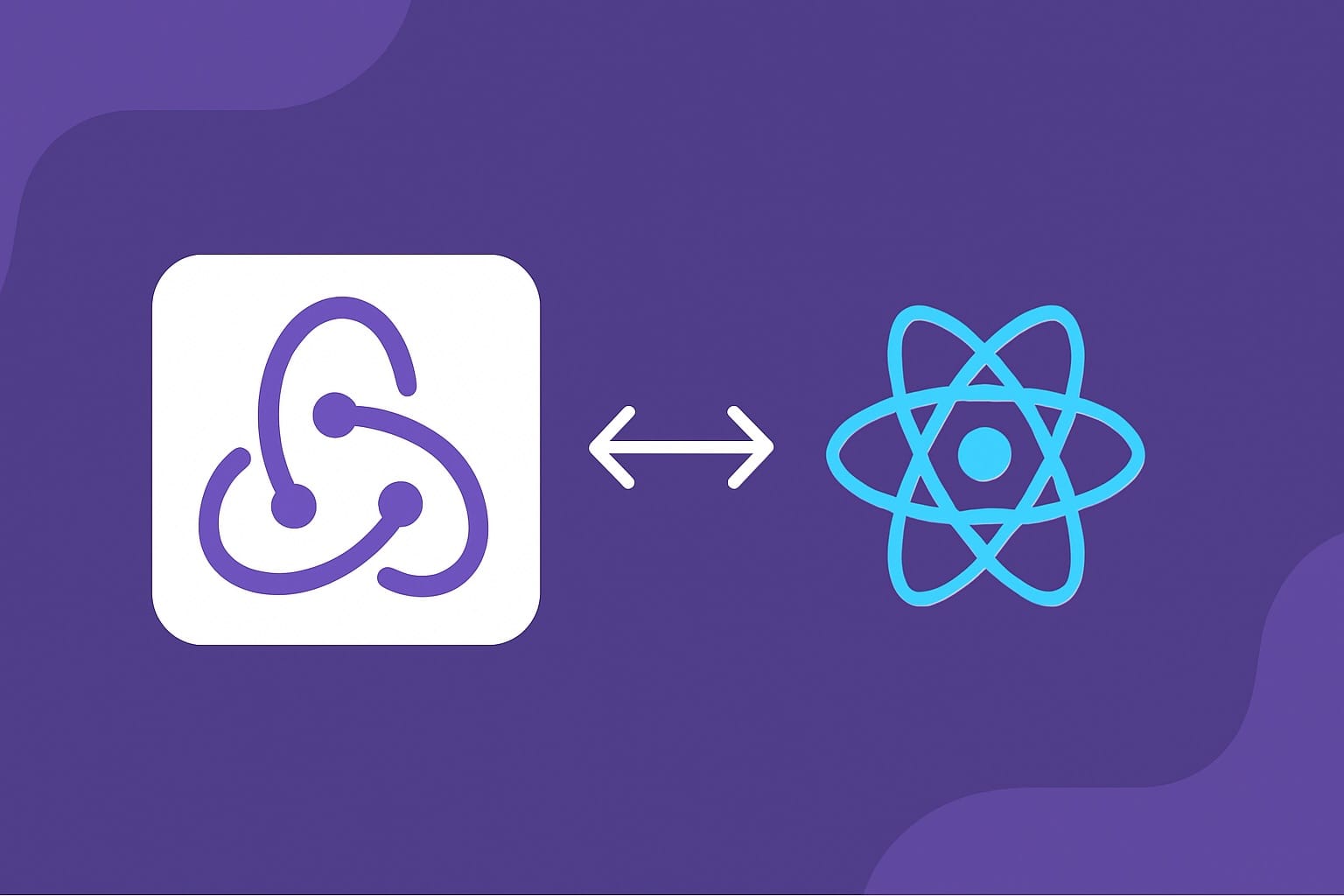
In this article, we'll cover Redux Toolkit fundamentals—its core concepts (like configureStore
and createSlice
), how it works, and why it's the standard for Redux development. Whether you're new or experienced, RTK is the best approach to Redux development. Check out this next article called Redux Toolkit Advanced: Scaling Redux Toolkit for Large Applications, where we'll dive into advanced topics like shared actions across slices, combined selectors and custom hooks.
What is Redux, BTW?
Before diving into Redux Toolkit, let’s quickly revisit Redux itself.
Redux is a state management library that helps us manage global state in JavaScript applications, especially but not only in React. It uses a centralised state management pattern, ensuring structured data flow and predictable state updates.
At its core, Redux is built around three main principles:
- Single Source of Truth: The global application state is stored in a single Redux store, making it easier to manage, debug, and track state changes throughout the app.
- State is Read-Only: The state cannot be modified directly. Instead, we dispatch actions to describe changes, ensuring that updates always follow a predictable and traceable flow.
- Changes are Made with Pure Functions: Instead of mutating state directly, reducers process actions and return a new state, ensuring predictable updates.
While Redux provides simple, but powerful state management, it requires a lot of boilerplate code and manual setup—which is where Redux Toolkit comes in to simplify things. Let’s see how! 🚀
Introducing Redux Toolkit (RTK)
Let’s briefly summarise key RTK concepts before exploring them in practice.
Redux Toolkit (RTK) is the official, recommended approach to writing Redux logic, designed to simplify development and minimize boilerplate.
In this article, we’ll dive into three basic core RTK concepts and use them to create a simple, functional ToDo list in React.
- configureStore: A wrapper around Redux’s
createStore
that simplifies store setup. It automatically combines slice reducers, includes redux-thunk by default (for asynchronous logic), enables the Redux DevTools Extension, and allows custom middleware. - createSlice: A higher-level abstraction that combines reducers and actions into a single API. It takes a slice name, an initial state, and an object of reducer functions, automatically generating the corresponding reducer, action creators, and action types.
- createEntityAdapter: normalizes data, generates reusable reducers, and improves performance by simplifying CRUD operations.
These are the main RTK concepts we’ll be using in this article as we explore how to implement them in practice. They simplify Redux development a lot and help manage state more efficiently. If you’d like to explore additional features, you can check the complete RTK API list in the official Redux Toolkit documentation.
Using Redux Toolkit in practice: Building a simple To-Do list
In this section, we'll explore how to use Redux Toolkit (RTK) in a practical scenario by building a simple to-do list application in React. From setting up a Redux store with configureStore
to creating slices with createSlice
and managing state efficiently, this guide will walk you through the key concepts of RTK. We'll also cover how to use createEntityAdapter
for optimized data handling and demonstrate how to dispatch actions and select state in a React app, all while keeping the code clean and minimal. 🚀
We'll follow this project structure:
📂 src
├── 📄 store.js
├── 📄 todoSlice.js
├── 📄 App.jsx
├── 📂 components
├────── 📂 TodoList
├───────── 📄 index.jsx
├───────── 📄 styles.css
Chek the this repo with all the files.
1. Install Dependencies
First, install the necessary dependencies:
npm install @reduxjs/toolkit react-redux
2. Setting Up the Redux Store
Before we create the reducers, we need to set up our Redux store using configureStore
from Redux Toolkit. Here, we define a todos
slice in the store, which will be managed by todoReducer
. This reducer will be created in the next step to handle actions like adding, toggling, and removing to-dos. Additionally, the slice name (todos) will be visible in the Redux DevTools, making it easy to track state changes during development.
// store.js
import { configureStore } from '@reduxjs/toolkit';
import todoReducer from './todoSlice';
export const store = configureStore({
reducer: {
todos: todoReducer,
},
});
3. Creating the To-Do Slice (Reducers & Actions)
In this step, we'll define the to-do slice using createSlice
, which generates reducers and actions for managing our to-do list. We'll start with a simple array-based approach, where each to-do item is stored as an object with id
, text
, and completed
properties. The reducers will handle adding, toggling, and removing to-dos.
Later, we'll improve this implementation by using createEntityAdapter
, which helps structure the state more efficiently by normalizing the data and generating optimized selectors and reducers. For now, we'll keep things simple and manage the list manually.
// todoSlice.js
import { createSlice } from '@reduxjs/toolkit';
// Initial state is an empty array, which will hold the to-do items
const initialState = [];
const todoSlice = createSlice({
// The name of the slice that will appear in the Redux DevTools
name: 'todos',
// The initial state of the slice
initialState,
// Reducers define how the state is updated based on dispatched actions
reducers: {
// Adds a new to-do to the list with a unique id, text, and a completed status (defaulted to false)
addTodo: (state, action) => {
state.push({
id: Date.now(), // Use the current timestamp as a unique id
text: action.payload, // The text for the new to-do item
completed: false, // New to-dos are not completed by default
});
},
// Toggles the completed status of a to-do item when it's clicked
toggleTodo: (state, action) => {
const todo = state.find((todo) => todo.id === action.payload); // Find the to-do by its id
if (todo) {
todo.completed = !todo.completed; // Toggle the completed status
}
},
// Removes a to-do item from the list by its id
removeTodo: (state, action) => {
return state.filter((todo) => todo.id !== action.payload); // Filter out the to-do to be removed
},
},
});
// Export the actions generated by createSlice
export const { addTodo, toggleTodo, removeTodo } = todoSlice.actions;
// Selector to get all to-dos from the store state
export const selectTodos = (state) => state.todos;
// Export the reducer function, which will be used in the store setup
export default todoSlice.reducer;
4. Building the To-Do List UI
In this step, we’ll create the user interface (UI) for our to-do list application using React. The UI will allow users to:
- Add new to-dos by typing into an input field and clicking the "Add" button.
- Toggle the completion status of a to-do by clicking on the task itself, which will mark it as completed or incomplete.
- Remove to-dos by clicking the delete (❌) button next to each item.
We'll use React hooks like useState
for local state and useDispatch
and useSelector
from react-redux
to interact with the Redux store. The UI will display a list of to-dos, and we'll conditionally apply styles to indicate whether a task is completed or not. The layout will be simple, and you can check the basic styling here.
Let’s get started by building the TodoList component!
import React, { useState } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { addTodo, toggleTodo, removeTodo, selectTodos } from '../../todoSlice';
import './styles.css'; // Import the CSS file
const TodoList = () => {
const [input, setInput] = useState(''); // Local state for the input field
const dispatch = useDispatch(); // Dispatch to trigger Redux actions
const todos = useSelector(selectTodos); // Select todos from the Redux store
// Handle adding a new todo
const handleAddTodo = () => {
if (input.trim()) {
// Check if input is not just whitespace
dispatch(addTodo(input.trim())); // Dispatch addTodo action
setInput(''); // Reset input field after adding the todo
}
};
// Handle toggling the completion status of a todo
const handleToggleTodo = (id) => {
dispatch(toggleTodo(id)); // Dispatch toggleTodo action to mark as completed or not
};
return (
<div className="todo-container">
<h1 className="todo-title">To-Do List</h1>
{/* Input field and Add button */}
<div className="input-container">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)} // Update input state on change
className="todo-input"
placeholder="Add a new task..."
/>
<button onClick={handleAddTodo} className="add-button">
Add
</button>
</div>
{/* List of todos */}
<ul className="todo-list">
{todos.map((todo) => (
<li
key={todo.id}
className={`todo-item ${todo.completed ? 'completed' : ''}`} // Add 'completed' class if the task is completed
>
{/* Checkbox to mark a task as completed or not */}
<label style={{ display: 'flex', alignItems: 'center' }}>
<input
type="checkbox"
checked={todo.completed} // Checkbox checked state based on todo's completed status
onChange={() => handleToggleTodo(todo.id)} // Handle checkbox toggle
className="todo-checkbox"
/>
<span
style={{
textDecoration: todo.completed ? 'line-through' : 'none',
}} // Strike-through text if completed
>
{todo.text}
</span>
</label>
{/* Remove button to delete a todo */}
<button
onClick={() => dispatch(removeTodo(todo.id))} // Dispatch removeTodo action
className="remove-button"
>
❌
</button>
</li>
))}
</ul>
</div>
);
};
export default TodoList;
Adicional explanations:
By using useSelector
, the component automatically subscribes to the Redux store and re-renders whenever the state it depends on (the list of todos
) changes. Similarly, useDispatch
provides the function needed to send actions to update the state in the store.
The selectTodos
function is a selector that returns the list of todos from the store, which is then used to display the tasks.
Using createEntityAdapter
for efficient state management
When managing collections of data in Redux, handling updates, deletions, and retrievals efficiently can become complex. Redux Toolkit’s createEntityAdapter
simplifies this process by normalizing data and providing optimized selectors and reducers out of the box.
Why Use createEntityAdapter
?
- Normalized State – Stores data in an object with IDs as keys instead of an array, making lookups and updates more efficient.
- Prebuilt Reducers – Includes
addOne
,addMany
,removeOne
,updateOne
, and more, reducing boilerplate code. - Auto-Generated Selectors – Provides optimized selectors (
selectAll
,selectById
, etc.) to retrieve entities easily.
Enhancing todoSlice.js
with createEntityAdapter
Now, we'll see how to improve the todoSlice.js
we previously created by using createEntityAdapter
. This approach will make our Redux state management more efficient, reducing boilerplate code and improving performance.
import { createSlice, createEntityAdapter } from '@reduxjs/toolkit';
// Create an adapter for the to-do list
const todosAdapter = createEntityAdapter();
// Define the initial state using the adapter's getInitialState
const initialState = todosAdapter.getInitialState();
// Create the slice
const todoSlice = createSlice({
name: 'todos', // The slice name will appear in Redux DevTools
initialState,
reducers: {
// Adds a new to-do using the adapter's addOne function
addTodo: (state, action) => {
todosAdapter.addOne(state, {
id: Date.now(), // Generate a unique ID based on timestamp
text: action.payload, // The text of the to-do
completed: false, // Default to not completed
});
},
// Toggles the completion status of a to-do item
toggleTodo: (state, action) => {
const todo = state.entities[action.payload]; // Access by ID
if (todo) {
todo.completed = !todo.completed; // Toggle completed status
}
},
// Removes a to-do using the adapter's removeOne function
removeTodo: (state, action) => {
todosAdapter.removeOne(state, action.payload);
},
},
});
// Export actions
export const { addTodo, toggleTodo, removeTodo } = todoSlice.actions;
// Generate selectors using the adapter
export const { selectAll: selectTodos, selectById: selectTodoById } =
todosAdapter.getSelectors((state) => state.todos);
// Export the reducer for the store setup
export default todoSlice.reducer;
Redux slice data without Adapter:
const state = {
todos: [
{
id: 1,
text: 'Buy groceries',
completed: false,
},
{
id: 2,
text: 'Finish project',
completed: true,
},
{
id: 3,
text: 'Call mom',
completed: false,
},
],
};
The todos
array stores objects, making it necessary to iterate over the array to find or modify items. To find a specific todo
, we need to use .find()
or .filter()
.
Updates require manually mapping through the array and modifying the correct item.
Redux slice data with Adapter:
const state = {
todos: {
ids: [1, 2, 3], // Stores the order of IDs
entities: {
1: { id: 1, text: 'Buy groceries', completed: false },
2: { id: 2, text: 'Finish project', completed: true },
3: { id: 3, text: 'Call mom', completed: false },
},
},
};
- Cleaner Code: Removes redundant logic.
- Better Performance: Direct lookups instead of filtering through an array.
- Easier Maintenance: Uses built-in Redux Toolkit methods.
Key Differences & Benefits of createEntityAdapter
Feature | Without createEntityAdapter |
With createEntityAdapter |
---|---|---|
State Structure | Array of objects | Normalized with entities |
Lookup Efficiency | O(n) (Loop through array) |
O(1) (Direct access via entities ) |
Adding a Todo | push new object |
addOne method |
Toggling Todo | Find by id then update |
Directly modify entities[id] |
Removing Todo | Filter out by id |
removeOne method |
Selectors | Manually written | Auto-generated (selectAll , selectById ) |
References
Conclusion
Redux Toolkit has transformed state management by simplifying Redux development and reducing boilerplate. With powerful utilities like configureStore
, createSlice
, and createEntityAdapter
, RTK enforces best practices while making state handling more efficient.
In this article, we explored the core concepts of Redux Toolkit and built a to-do list to demonstrate its benefits. We also compared manual state management with createEntityAdapter
, highlighting how it normalizes data, improves performance, and simplifies CRUD operations.
By adopting Redux Toolkit, developers can write cleaner, more maintainable Redux code with minimal setup. If you're still managing state the old way, now is the perfect time to embrace RTK for a better development experience! 😃
See you in the next article!