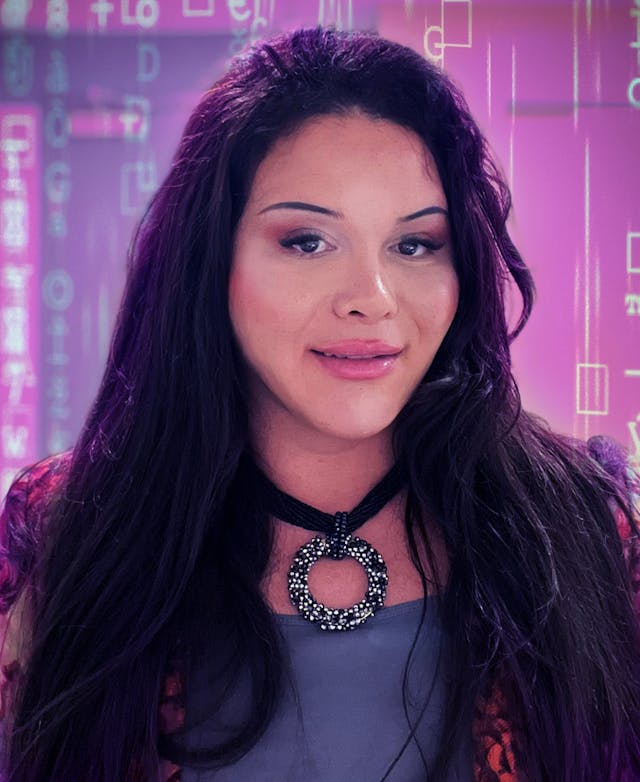
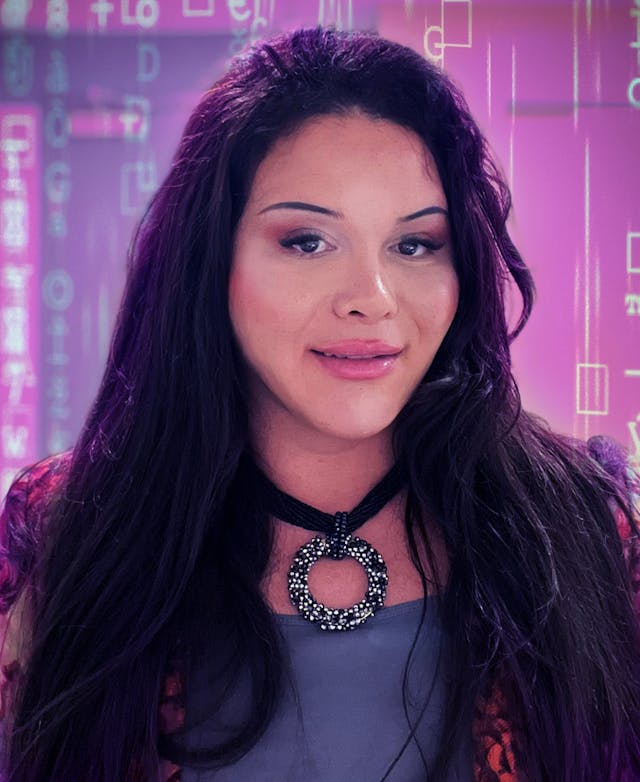
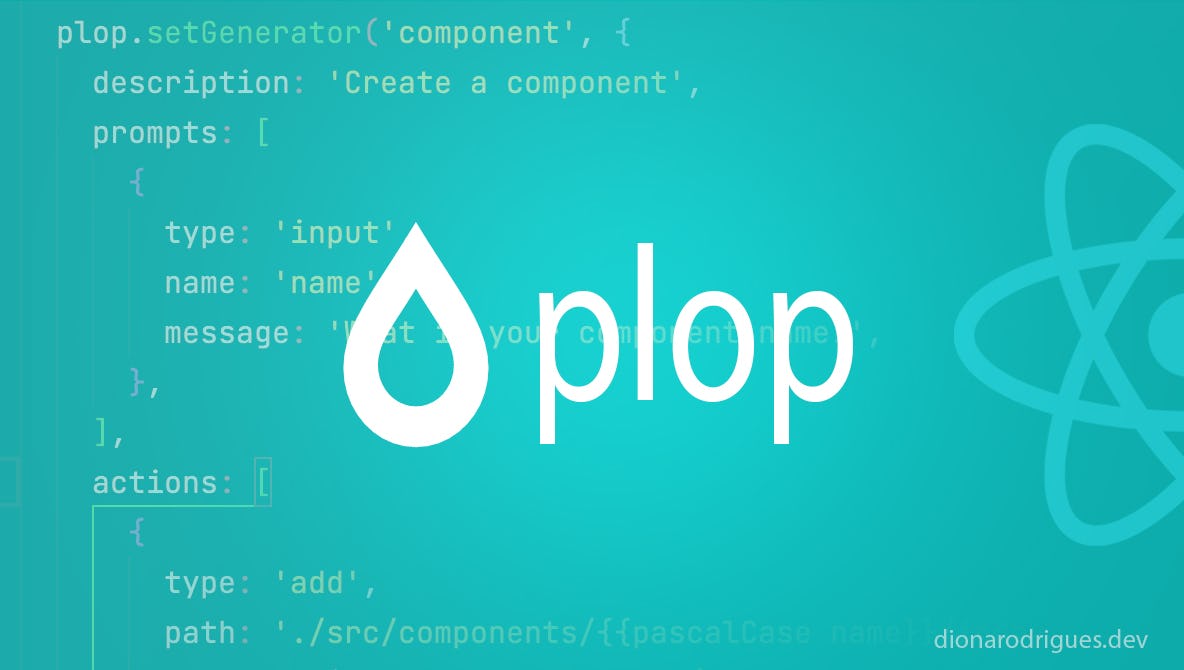
I've had the opportunity to work on several large projects for different companies and certainly maintaining code consistency among developers is one of the most challenging tasks I've ever seen - even working on some personal projects, how many times have I lost the consistency of my code? Having good documentation and code reviews are always awesome solutions, but what if we had a tool to help us with this, automating some trivial steps? Plop might be one of the greatest way to handle it.
What’s Plop?
"Plop is a little tool that saves you time and helps your team build new files with consistency." - Plop
In short, Plop is a node dependency that we can install in our projects, and using a single command line, it can generate files following a pre-defined structure, such as React components or pages, styles, tests... But not just it, by using Plop we can also define some template for these files, isn’t it awesome?
How to use Plop in React Projects
As Plop is a dependency of Node you can use it not only with React, but also with other libraries and frameworks as well, however for this mini tutorial we will only focus on React, which is the subject of this article. 😁
That said, Below you will learn how to install Plop in a project, configure it and generate components and pages using the terminal in 5 steps. And for a better understanding you can check all files in this Github repo. Let's go?
1- Setting up a quick React Project
If you don’t have a started React project which you can use to test Plop, all you have to do is:
- In your terminal, create a new React project by using Create React App:
npx create-react-app my-app
.
2- Installing Plop
Assuming you already have a React project and your terminal is pointed to the project directory:
- run the following command line to install the Plop dependency in your project:
npm install --save-dev plop
- setup an npm script in
package.json
to be able to run Plop from the terminal:
// package.json
"scripts": {
…
"plop": "plop"
},
Later, with this config, you’ll be able to run npm run plop
from the terminal.
3- Defining a template for components and pages
Before we configure Plop to generate the files for us, let's create a simple template to inject some code into the files when creating components and pages. This is optional and you can have different templates, but here we'll just use one to simplify this tutorial.
Templates for Plop are created using Handlebars, which is why their file extension is .hbs
.
- In the root directory, create a folder called
plop-templates
(can be any name) - Inside this folder create a file called
component.hbs
- Copy and paste the following code into this file:
// ./plop-templates/component.hbs
import './styles';
function {{pascalCase name}}() {
return (
<div>
<h1>{{pascalCase name}}</h1>
</div>
);
}
export default {{pascalCase name}};
If you’re using Prettier to format your files on save command, maybe you’ll need to disable it for .hbs
files. So, open or create a file called .prettierignore
in the root directory of your project and into there: /plop-templates/**/*.*
.
As you can see, this template contains an import to its CSS file along with the component function declaration and its default export. The component name will also be transformed into pascal syntax when generating the files using Plop, thus, the word “main button” will be transformed into “MainButton”.
4- Creating the Plop file
After installing the Plop dependency and creating the template, follow the steps below to configure Plop to generate the files in your React project:
- In the root directory, create a file called
plopfile.js
- Copy and paste the following code into this file:
module.exports = (plop) => {
// ./plopfile.js
// Setting up Plop to generate components
// When run `npm run plop` you'll be asked to enter the component name.
plop.setGenerator('component', {
description: 'Create a component',
prompts: [
{
type: 'input',
name: 'name',
message: 'What is your component name?',
},
],
actions: [
{
type: 'add',
path: './src/components/{{pascalCase name}}/index.js',
templateFile: 'plop-templates/component.hbs',
},
{
type: 'add',
path: './src/components/{{pascalCase name}}/styles.css',
},
],
});
// Setting up Plop to generate pages
// When run `npm run plop` you'll be asked to enter the page name.
plop.setGenerator('page', {
description: 'Create a page',
prompts: [
{
type: 'input',
name: 'name',
message: 'What is your page name?',
},
],
actions: [
{
type: 'add',
path: './src/pages/{{pascalCase name}}/index.js',
templateFile: 'plop-templates/component.hbs',
},
{
type: 'add',
path: './src/pages/{{pascalCase name}}/styles.css',
},
],
});
};
As you can see we configured Plop to generate components and pages. Therefore, every time you run npm run plop
, you will first need to choose between one of these two options and then enter a name for it. In the prompt
section we configure the question to appear in the terminal when running Plop while in the action
section we define the files that Plop will generate, where they will be placed and the optional template to be used to inject some initial code into these files. Here we are using the same template for components and page files, but it can be different of course. For styles, we are not using any templates, just creating an empty CSS file every time Plop is run.
See more ways to configure this file in the Plop documentation.
5- Generating the files by using terminal
Once all above steps are done, all you need to do is:
- With the terminal configured in the project root directory, run
npm run plop
- Then choose between “component” or “page”
- Enter the name of it
- Check the generated files in
./src/components
or./src/pages
depending on what you chose in step 2
Easy peasy, isn’t it? I hope you learned how to configure Plop and how it is beneficial for your projects.
Conclusion
Plop is a great alternative to avoid repetitive manual steps every time we need to create new components and pages in React. It provides us with a way to define where these files will be placed and also a template for each of them using just a single command line in the terminal, after some quick config.
And especially for large projects with many developers involved, Plop is also an amazing way to maintain code consistency over time, which is a very challenging task in software development.
I hope you learned how to add and use Plop in your projects. Let me know if you've heard of or used Plop before.
See you next time! 😁